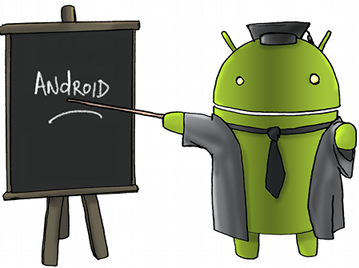
Best Practices for Background Jobs
These classes show you how to run jobs in the background to boost your application’s performance and minimize its drain on the battery.
Running in a background service
This class describes how to implement an IntentService, send it work requests, and report its results to other components.
Creating a background service
The IntentService class provides a straightforward structure for running an operation on a single background thread. This allows it to handle long-running operations without affecting your user interface’s responsiveness. Also, an IntentService isn’t affected by most user interface lifecycle events, so it continues to run in circumstances that would shut down an AsyncTask.
An IntentService has a few limitations:
- It can’t interact directly with your user interface. To put its results in the UI, you have to send them to an Activity.不能直接与UI交互
- Work requests run sequentially. If an operation is running in an IntentService, and you send it another request, the request waits until the first operation is finished.任务顺序执行
- An operation running on an IntentService can’t be interrupted.任务不可被打断
However, in most cases an IntentService is the preferred way to simple background operations.