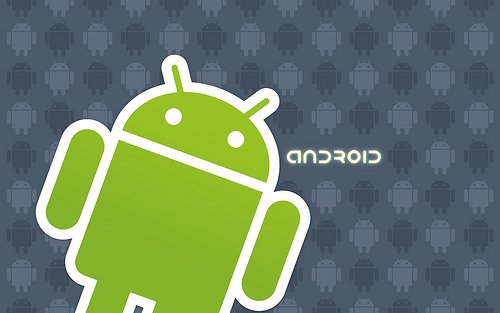
I originally wrote this article when I was (foolishly) still using AsyncTasks. Nowadays I simply consider it a mistake in all cases. As you’ll see from the original article, there are a lot of problems with it – and there are much better solutions.
My preferred alternative these days are combining RxJava with schedulers. You get the same effect as an AsyncTask
with none of the problems, plus you get an awesome framework in addition. I know, recommending a library to solve a problem is irritating, but RxJava is worth looking at for many reasons.
When AsyncTask
was introduced to Android, it was labeled as “Painless Threading.” Its goal was to make background Threads which could interact with the UI thread easier. It was successful on that count, but it’s not exactly painless – there are a number of cases where AsyncTask
is not a silver bullet. It is easy to blindly use AsyncTask
without realizing what can go wrong if not handled with care. Below are some of the problems that can arise when using AsyncTask
without fully understanding it.